If I create a BOX primitive in my existing project A, PlayCanvas | HTML5 Game Engine it creates a visible box with a RENDER component only. No other components.
If I create a BOX primitive in my new project B, PlayCanvas | HTML5 Game Engine it creates a visible box with a MODEL component only. No other components.
Why is there a difference between my two projects? This has a very negative effect, specifically I cannot switch materials on my primitive with a “RENDER” component. Switching materials on my project B is no problem.
Thanks
Charlie
UPDATE 1* It appears RENDER component is a beta feature with a planned replacement of MODEL component. But I don’t want this feature in my project, as it prevents me from changing materials on my primitive cube. How can I turn it off? Render vs Model Component?
I made a 30 second screen capture to illustrate the difference
Just to help clarify a few things here:
-
Render component is no longer beta. Going forward, we will be looking to deprecate Model and Animation component and replace it with Render and Anim component.
-
To disable the preset of primitive to Render, disable ‘Import Hierarchy’ in the Asset tasks. (Note that Import Hierarchy uses the Render Component when importing FBXs)
-
Or you can build the primitive from scratch by creating an Entity and adding a Model Component
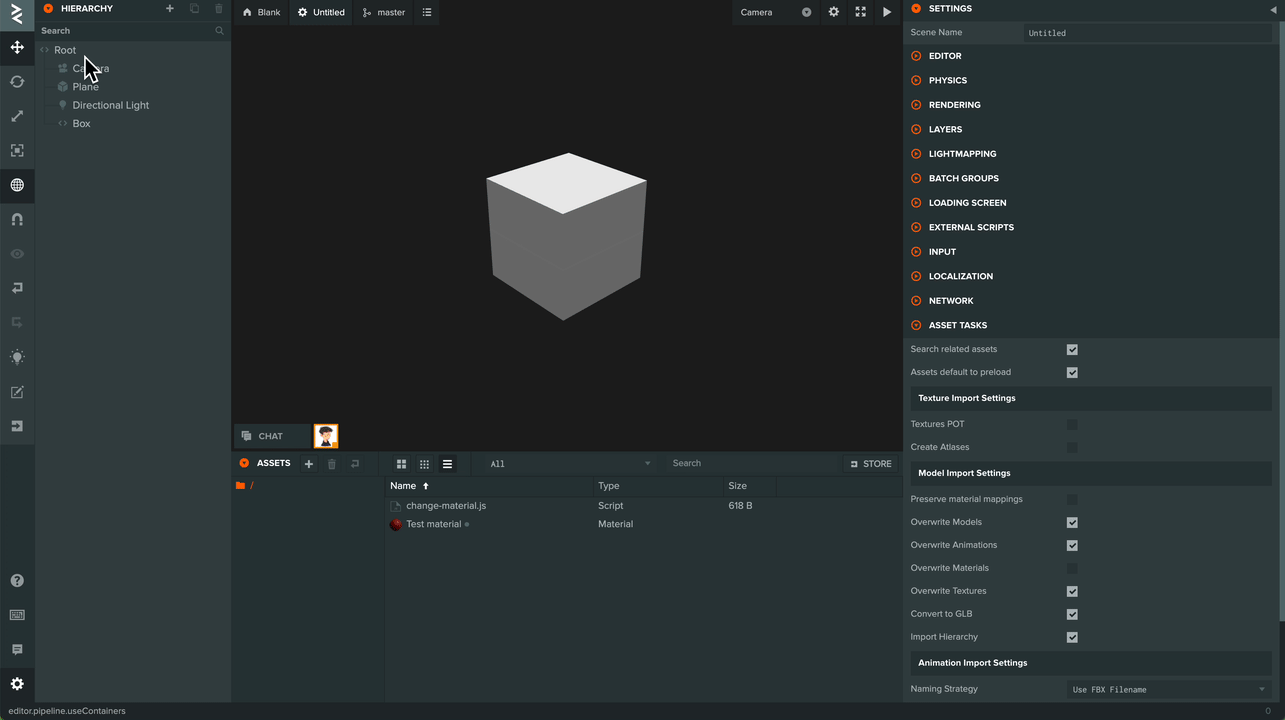
-
To change the material on a primitive on a Render component, use the material
property RenderComponent | PlayCanvas API Reference
// initialize code called once per entity
ChangeMaterial.prototype.initialize = function() {
var matAsset = this.app.assets.find('Test material', 'material');
this.entity.render.material = matAsset.resource;
};
Project example: https://playcanvas.com/editor/scene/1183667
I see you are using the script from the tutorials for the model component: Switching materials at runtime | Learn PlayCanvas
You need to change the code so that it accesses the Render component instead.
// More information about materials: http://developer.playcanvas.com/en/tutorials/beginner/basic-materials/
var SwitchingMaterials = pc.createScript('switchingMaterials');
// Create an array of materials to cycle the model through
SwitchingMaterials.attributes.add("materials", {type: "asset", assetType: "material", array: true, title: "Materials"});
// initialize code called once per entity
SwitchingMaterials.prototype.initialize = function() {
var self = this;
this.materialIndex = 0;
// Change materials every second
setInterval(function () {
self.changeToNextMaterial();
}, 1000);
};
SwitchingMaterials.prototype.changeToNextMaterial = function(dt) {
// Get the next material asset in the array
this.materialIndex = (this.materialIndex + 1) % this.materials.length;
var material = this.materials[this.materialIndex];
// Assign the material to all the mesh instances in the model
var meshInstances = this.entity.render.meshInstances;
for (var i = 0; i < meshInstances.length; ++i) {
var mesh = meshInstances[i];
mesh.material = material.resource;
}
};
Thanks @yaustar ! I changed import settings and it’s working as expected. I’ll give the entity.render.meshInstances a try as well.