this is the project i forked
https://playcanvas.com/editor/project/774862
In this project you are spawning some plants (as many as you give the screen).
I would like to know if there is any way to spawn only one object and disable the option to put more objects.
Thank you very much and greetings to all. (sorry for my english which is not very good)
Hi @RafaelLopera,
That’s definitely doable, there is a number of ways you can do that change.
The easiest way looking at the hit-test.js
script is to update the pointerUpdated
property only once, that will have the effect of placing only a single object in the lifetime of the application.
// update line 30 to:
if(self.pointerUpdated === 0){
self.pointerUpdated = 1;
}
update line 30 as you told me, but I keep putting objects when I press click,
Ah right, I see that counter is being reset at the bottom of the script. Nevermind I’ve updated your script to included a maxCount property, set it to whatever you like e.g. 1 to control the max number of spawn objects.
var HitTest = pc.createScript('hitTest');
HitTest.attributes.add('object', { type: 'asset', assetType: 'template' });
HitTest.attributes.add('pointer', { type: 'entity' });
HitTest.attributes.add('maxCount', { type: 'number', default: 1, min: 0, });
// initialize code called once per entity
HitTest.prototype.initialize = function() {
if (! this.app.xr.hitTest)
return;
var self = this;
this.countCreated = 0;
this.lastCreated = 0;
this.pointerUpdated = 0;
// when XR session is started
this.app.xr.on('start', function() {
// start hit test from viewer position (middle of the screen)
this.app.xr.hitTest.start({
spaceType: pc.XRSPACE_VIEWER,
callback: function(err, hitTestSource) {
// when hit test session started
hitTestSource.on('result', function(position, rotation) {
// position and rotate pointer to hit point
self.pointer.setPosition(position);
self.pointer.setRotation(rotation);
// enable pointer
self.pointer.enabled = true;
// set pointer state to 1
self.pointerUpdated = 1;
self.app.fire('hitTestAvailable');
});
}
});
});
};
HitTest.prototype.update = function() {
if( this.countCreated >= this.maxCount ) return;
// if pointer been updated
if (this.pointerUpdated === 1) {
// set pointer state to 2, which will hide pointer next frame
this.pointerUpdated = 2;
// if user taps on screen (input source is available)
if (this.app.xr.input.inputSources.length) {
var now = Date.now();
// every 200 milliseconds
if ((now - this.lastCreated) > 200) {
this.lastCreated = now;
// clone object
var ent = this.object.resource.instantiate();
ent.enabled = true;
// position on picked location
ent.setPosition(this.pointer.getPosition());
// rotate randomly
ent.setEulerAngles(0, Math.random() * 360, 0);
// scale a bit randomly
ent.setLocalScale(Math.random() * 0.5 + 0.3, Math.random() * 0.3 + 0.3, Math.random() * 0.5 + 0.3);
ent.reparent(this.app.scene.root);
this.countCreated++;
}
}
} else if (this.pointerUpdated === 2) {
// if pointer state is 2, reset state
this.pointerUpdated = 0;
// and hide pointer
this.pointer.enabled = false;
}
};
After parsing the script in editor:
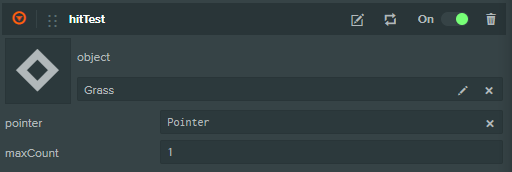
Tested on Chrome with the Mozilla WebXR emulator extension:
1 Like
Thank you very much, it works perfectly, now I will be implementing my things. All the best
1 Like