Here, I want to use json file that I will define tiletype names, resource types, and resource names, and instructions to generate each tile type with max amount for each resource. I am not sure how to use a json file in a script, as I have never used a json file before. Anyone have any ideas? (I need the script to somehow read the json file, and find names in editor for tiletypes and resources)
Here is the json file:
{
"tileTypes": ["grass", "dirt", "sand", "mountain"],
"resourceTypes": {
"trees": {
"resources": ["tree1", "cactus1"]
},
"minerals": {
"resources": ["rock1", "rock2", "metal2"]
},
"ediblePlants": {
"resources": ["mushroom1"]
}
},
"generateForChunks": {
"grass": {
"resources": {
"tree1": {"max": 5},
"mushroom1": {"max": 3}
}
},
"dirt": {
"resources": {
"rock1": {"max": 10},
"rock2": {"max": 7}
}
},
"sand": {
"resources": {
"cactus1": {"max": 15}
}
},
"mountain": {
"resources": {}
}
}
}
Here is how you can read the contents of your json file. Assuming that it is preloaded.
let config = this.app.assets.find('config.json').resource;
console.log(config.resourceTypes);
console.log(config.resourceTypes.trees);
console.log(config.resourceTypes.trees.resources[0]);
console.log(config.resourceTypes.minerals);
console.log(config.resourceTypes.minerals.resources[0]);
console.log(config.generateForChunks);
console.log(config.generateForChunks.grass.resources.tree1.max);
1 Like
But how can I let’s say use a script to read the json file, then find each entity in tile types and resource types in the editor by name?
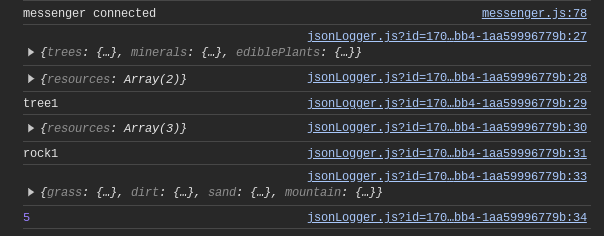
I did some testing for logging it, it works so thank you, I will try to use the values to build the terrain based on the information, here is the script if you have any suggestions:
var JsonLogger = pc.createScript('jsonLogger');
// Define an attribute on the script that points to the JSON asset
JsonLogger.attributes.add('configJson', { type: 'asset', assetType: 'json', title: 'Config JSON' });
// initialize code called once per entity
JsonLogger.prototype.initialize = function() {
if (!this.configJson) {
console.error('The config JSON asset is not set.');
return;
}
// Load the asset if it's not already loaded
if (!this.configJson.resource) {
this.app.assets.load(this.configJson);
this.configJson.ready((asset) => {
this.logJson(asset.resource);
});
} else {
// Asset is already loaded, directly log its contents
this.logJson(this.configJson.resource);
}
};
// Function to log specific parts of the JSON data
JsonLogger.prototype.logJson = function(config) {
console.log(config.resourceTypes);
console.log(config.resourceTypes.trees);
console.log(config.resourceTypes.trees.resources[0]);
console.log(config.resourceTypes.minerals);
console.log(config.resourceTypes.minerals.resources[0]);
console.log(config.generateForChunks);
console.log(config.generateForChunks.grass.resources.tree1.max);
};
// Make sure to attach this script to an entity and set the 'configJson' attribute
// to point to your 'config.json' asset in the PlayCanvas editor.
I have the working code, it looks nice, and I use the findByName to make worlds based off of the json instructions. Here is a screenshot of the world generated with seed: 0 (I am at x: -101, z: -44)
I am now working on saving worlds with json file, and loading worlds as well. That being said I do appreciate your help with the scripting as I am completely new to json files.
1 Like