When we upload fbx into playcanvas studio it creates a separate glb for an animation.
Is there any way I can use those separate animation glb for the source glb?
According to playcanvas engine example, we need animStateGraphData to use those animation glb.
Is it possible to use without animStateGraphData?
Can I simply apply the animation glb into its source glb?
What do you mean by separate animation GLB? Do you mean by uploading a GLB file to the Editor?
Yes. Once an fbx with animation is uploaded, two glb files are craeted.
One is for model and the other one is for animation.
Sorry, I still don’t understand what you mean here:
Is there any way I can use those separate animation glb for the source glb?
What do you mean by source GLB?
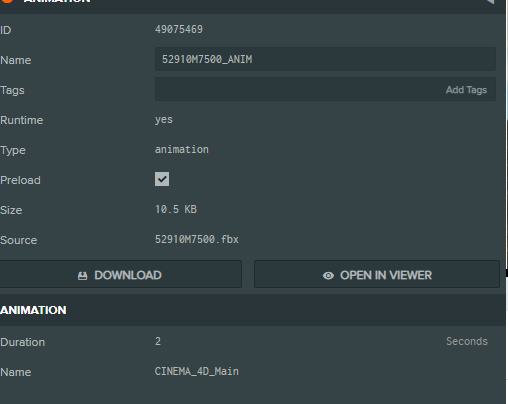
If you see the capture image, animation type has its source.
The source is also converted an glb file itself.
I want to download all of them and use it only with playcanvas engine.
Ah, I see. The source is more for the Editor so that it knows when you upload that FBX again, it updates the GLB assets. It doesn’t affect the engine runtime at all.
According to playcanvas engine example, we need animStateGraphData to use those animation glb.
You don’t have to use the anim state graph, you can still use the old Animation Component instead with the GLB assets.
When I upload 52190M7500.fbx file into the studio, it creates 52190M7500.glb for model and another 52190M7500.glb for animation.
As far as I understand, the first one (52190M7500.glb) doesn’t have animation clip data. but the second one( 52190M7500.glb for animation) has all the information for animation. Is it correct??
According to the api document https://developer.playcanvas.com/en/api/pc.AnimComponentLayer.html#assignAnimation
the method assignAnimation is part of AnimComponentLayer and it seems like the assignAnimation is related to anim state graph.
Is there any simpler way to use those model glb and animation glb?
I used to check if the model has animation key frame as the below way.
this.frontLeftDoorFrameCnt = entity.animComponent.animClips[0].animCurves[0].animKeys.length;
I’m trying to merge model the model glb and the animation glb as below.
// Load a model file and create a Entity with a model component
var url = "../assets/models/52910M7500.glb";
var aniUrl = "../assets/models/52910M7500_ANIM.glb";
app.assets.loadFromUrl(url, "container", function (err, asset) {
app.start();
var entity = new pc.Entity();
entity.addComponent("model", {
type: "asset",
asset: asset.resource.model,
castShadows: true
});
console.log(entity, entity.animation);
app.assets.loadFromUrl(aniUrl, "animation", function (err, animAsset) {
console.log(animAsset);
entity.addComponent("anim", {
activate: true,
asset: animAsset._resource,
speed: 2,
playing: true
});
console.log(entity);
app.root.addChild(entity);
// Create an Entity with a camera component
var camera = new pc.Entity();
camera.addComponent("camera", {
clearColor: new pc.Color(0.4, 0.45, 0.5)
});
camera.translate(0, 0, 3);
app.root.addChild(camera);
// Create an entity with a directional light component
var light = new pc.Entity();
light.addComponent("light", {
type: "point"
});
light.setLocalPosition(1, 1, 5);
app.root.addChild(light);
});
// app.on("update", function (dt) {
// if (entity) {
// entity.rotate(0, 10 * dt, 0);
// }
// });
});
</script>
After I added “anim” component as an animation to the entity with the resource of the animation, I checked the object detail of entity but I couldn’t find animClips from any level from the object.
Is there anything I missed?
Sounds like it be easier to use the AnimationComponent. Example of creating it at runtime: https://playcanvas.com/editor/scene/1172259
App.prototype.initialize = function() {
var app = this.app;
var url = "https://raw.githubusercontent.com/yaustar/yaustar.github.io/master/test-anim-glbs/male.glb";
var aniUrl = "https://raw.githubusercontent.com/yaustar/yaustar.github.io/master/test-anim-glbs/Idle%20(3).glb";
app.assets.loadFromUrl(url, "container", function (err, asset) {
var entity = new pc.Entity();
entity.addComponent("model", {
type: "asset",
asset: asset.resource.model,
castShadows: true
});
app.assets.loadFromUrlAndFilename(aniUrl, "idle.glb", "animation", function (err, animAsset) {
entity.addComponent("animation", {
activate: true,
assets: [animAsset],
});
app.root.addChild(entity);
});
});
};
Thanks @yaustar
After refering your code, I tried to activate the animation later, but somehow enabling activation property is not working as expected.
entity.addComponent("animation", {
assets: [animAsset],
activate: false,
});
app.root.addChild(entity);
var light = new pc.Entity();
light.addComponent("light", {
type: "point"
});
light.setLocalPosition(1, 1, 5);
app.root.addChild(light);
entity.animation.activate = true;
Basically what I’m trying to archive is activate the animation later and move to a specific time frame based on UI event.
How come, even activating animation is not working.???
The activate property is only used when the component is added to the entity: https://developer.playcanvas.com/en/api/pc.AnimationComponent.html#activate
After it’s been added, it has no effect.
Use play() to play the animation if you want the animation to play later. https://developer.playcanvas.com/en/api/pc.AnimationComponent.html#play
Example with timeout:
// initialize code called once per entity
App.prototype.initialize = function() {
var app = this.app;
var url = "https://raw.githubusercontent.com/yaustar/yaustar.github.io/master/test-anim-glbs/male.glb";
var aniUrl = "https://raw.githubusercontent.com/yaustar/yaustar.github.io/master/test-anim-glbs/Idle%20(3).glb";
var entity;
app.assets.loadFromUrl(url, "container", function (err, asset) {
entity = new pc.Entity();
entity.addComponent("model", {
type: "asset",
asset: asset.resource.model,
castShadows: true
});
app.assets.loadFromUrlAndFilename(aniUrl, "idle.glb", "animation", function (err, animAsset) {
entity.addComponent("animation", {
activate: false,
assets: [animAsset],
});
app.root.addChild(entity);
});
});
setTimeout(function() {
entity.animation.play('idle.glb', 0);
}, 3000);
};
https://playcanvas.com/editor/scene/1172259