I want to use tweet.js to move the orbitCamera to a place. The camera can be moved, but the angle does not rotate. SetPosition and setRotation seem to have no effect.After I use setPosition, the camera will return to its original position,this is strange
GetCameraData.prototype.useTween = function () {
var that = this;
var currentPos = this.camera.getPosition().clone();
var targetPos = this.targetEntity.getPosition().clone();
var alpha = 0;
var tween = this.app.tween(alpha).to(1, 3, pc.SinIn);
tween.on("update", function (dt) {
currentPos.lerp(currentPos, targetPos, dt * 2);
// this.camera.setPosition(currentPos.x, currentPos.y, currentPos.z);
this.camera.script.orbitCamera.pivotPoint = currentPos;
}, this);
tween.start();
};
Through the pivot point of orbitCamera, I can move to a specific place and stop. This is the effect I want. What is a good way to do rotation?
project link:PlayCanvas | HTML5 Game Engine
As you know, there is an API and functions to move the camera.
You can move the pivotPoint
to pan the camera and change the point of orbit
distance
for the distance between the pivot and the camera
yaw
and pitch
But there’s also a few more functions that you can find in the code: PlayCanvas | HTML5 Game Engine
The one that is probably the most useful to you is the resetAndLookAtPoint
which allows you to change the pivot point and position of the camera in a single call.
Sorry, I didn’t see your reply because of the holiday. I tried the resetAndLookAtPoint method, which allows my orbitCamera to move to and look at the target object, but the effect between moving is a little strange.Did I use it wrong?
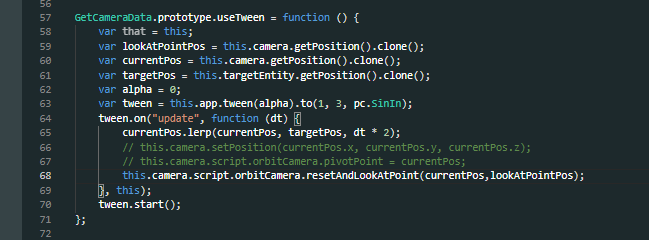
The camera is not slowly turning to the object
I looked at the code again. It seems that I used it wrong

Now I can move to a specific location.
Another question is, can orbitCamera use this camera path?I use orbitCamera script directly on camera path, but it is not easy to use
Should be pretty straightforward. If you look at code for the camera path, it sets the camera position and the look at point.
The look at point is the same thing as the pivot point on the orbit camera.
I’m not very good at using camera path code,I move the camera through the curve, which can be achieved.Does this method consume performance or something?
if (that.time > that.duration) return;
var percent = that.time / that.duration;
var curveValue = that.posCurve.value(percent);
var lookAtValue = that.lookAtCurve.value(percent);
// console.log(curveValue);
that.curvePosition.x = curveValue[0];
that.curvePosition.y = curveValue[1];
that.curvePosition.z = curveValue[2];
that.lookAtPosition.x = lookAtValue[0];
that.lookAtPosition.y = lookAtValue[1];
that.lookAtPosition.z = lookAtValue[2];
that.camera.script.orbitCamera.resetAndLookAtPoint(that.curvePosition, that.lookAtPosition);
}
What’s the context? It shouldn’t do anything that would be noticeable on framerate etc
https://playcanvas.com/editor/code/1035705?tabs=120373166
var GetCameraData = pc.createScript('getCameraData');
GetCameraData.attributes.add('camera', {
type: 'entity'
});
GetCameraData.attributes.add('targetEntity', {
type: 'entity'
});
GetCameraData.attributes.add('entity1', {
type: 'entity'
});
GetCameraData.attributes.add('currentPoint', {
type: 'entity'
});
GetCameraData.attributes.add('posCurve', {
type: 'curve',
curves: ['x', 'y', 'z']
});
GetCameraData.attributes.add('lookAtCurve', {
type: 'curve',
curves: ['x', 'y', 'z']
});
GetCameraData.attributes.add("duration", {
type: "number",
default: 3
});
// initialize code called once per entity
GetCameraData.prototype.initialize = function () {
var that = this;
this.time = 0;
this.app.addTweenManager();
this.curvePosition = new pc.Vec3();
this.lookAtPosition = new pc.Vec3();
this.curveState = false;
this.entity.button.on('click', function () {
// that.cameraData();
this.curveState = true;
}, this);
};
// update code called every frame
GetCameraData.prototype.update = function (dt) {
var that = this;
this.time += dt;
if (this.app.keyboard.wasPressed(pc.KEY_1)) {
// this.useTime();
// this.useTween();
this.curveState = true;
}
if (this.curveState == true) {
if (that.time > that.duration) return;
var percent = that.time / that.duration;
var curveValue = that.posCurve.value(percent);
var lookAtValue = that.lookAtCurve.value(percent);
// console.log(curveValue);
that.curvePosition.x = curveValue[0];
that.curvePosition.y = curveValue[1];
that.curvePosition.z = curveValue[2];
that.lookAtPosition.x = lookAtValue[0];
that.lookAtPosition.y = lookAtValue[1];
that.lookAtPosition.z = lookAtValue[2];
that.camera.script.orbitCamera.resetAndLookAtPoint(that.curvePosition, that.lookAtPosition);
}
};
// swap method called for script hot-reloading
// inherit your script state here
// GetCameraData.prototype.swap = function(old) { };
// to learn more about script anatomy, please read:
// https://developer.playcanvas.com/en/user-manual/scripting/
I want to use a lot of points in the curve. I don’t know if it will cause the main page to get stuck?