Hello, my goal is to manipulate all the children of a selected entity using an editor script. Messing around with what I could see in the console and the api documentation, I got to this point.
let selected = editor.selection.items[0];
The following code doesn’t work because “set” is not a function:
let children = selected.viewportEntity.children;
for (let i = 0; i < children.length; i++)
{
children[i].set(“localPosition”, {x: 0, y: 0, z: 0});
children[i].set(“localScale”,{x: 1, y: 1, z: 1});
}
The following code affects a console log of the entity, but doesn’t change anything in the editor:
let children = selected.viewportEntity.children;
for (let i = 0; i < children.length; i++)
{
children[i].localPosition = {x: 0, y: 0, z: 0};
children[i].localScale = {x: 1, y: 1, z: 1};
}
I’m pretty sure there’s just a function I need to call or event to fire to tell the editor that I’ve updated something, but I don’t know where I’d look for that. My other theory is that the entities in “viewportEntity” are only for UI and don’t affect the functionality.
Does anyone have an insights here?
Hi @Christopher_Poole, can you share a sample project? It is difficult to understand what editor.selection is.
Also, we cannot set vector 3 like this {x, 0, y: 0, z: 0}.
looking at the Entity API here: Entity | PlayCanvas API Reference
it seems that this should work with .set
:
also, .children
does not give you entities directly, but resource_id’s of the entity:
Sorry if this wasn’t clear, but this is using the Editor API so its a script I’m loading on the webpage using ViolentMonkey following this tutorial: Editor API | PlayCanvas Developer Site
This is where I got the idea to use “.set” but like I said that gives the error: TypeError: children[i].set is not a function
Yes, that was clear to me, and that’s why I posted links to an Editor API.
Oh totally my bad, I didn’t look carefully enough at your link.
The issue was that I’d gotten a list of viewportEntities not Entities.
I was initially confused because when you console.log an Entity you get a fairly barebones set of outputs:
(From the Editor API example)
const entities = editor.entities.root.listByTag(‘red’);
for (const entity of entities) {
console.log(entity);
}
returns
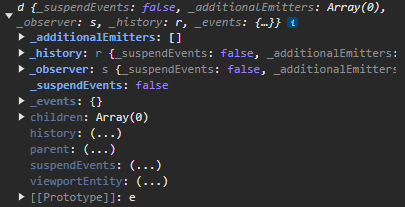
vs when you console.log a viewportEntity
With the help of the documentation you linked, I was able to set and get values without needing to see what was in the console.log.
My modified code removes “viewportEntities” from the selection code, because I realized after comparing the two console.logs that selection returns and Entity itself.
let children = selected.children;
for (let i = 0; i < children.length; i++)
{
children[i].set(“position”, [0, 0, 0]);
children[i].set(“scale”, [1, 1, 1]);
}
Thanks for the help!
1 Like
Slight correction: Set the position and scale via an array not an object
box.set(‘position’, [x, y, z]);
1 Like