I would like to learn ways I could implement the UI screen using the 2D Screen system of the engine. The game can be run on both PC browsers and phone devices, so ideally it should scale and maintain relative position of the elements accordingly.
Here is my current Home Screen view of the game, which uses my current attempt:
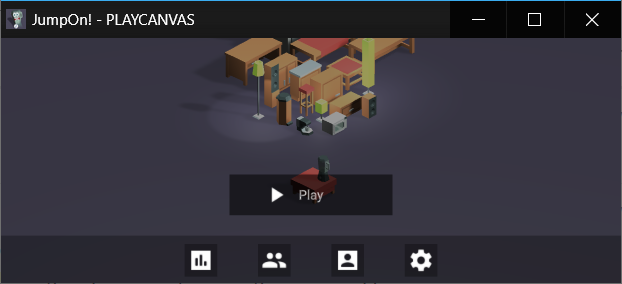
The bottom tint, is a semi-transparent Image Element, which I use as a footer. You can see the hierarchy here:
That footer background is set so that it is always at the bottom and takes 20% of the screen height:
The buttons use that footer tint as a parent to position themselves on the screen. They simply try to be at the center of it. They are all grouped inside a Group Element, which has a Group Layout component. This makes them align themselves horizontally and evenly space out from each other. I placed the Play button as a child of that footer too and simply offset it higher, so it is always at the same position relative to the footer, no matter the screen size.
This works well on mobile devices, no matter how I orient the device. However, I am facing an issue of properly scaling it on a PC monitor. The screenshot will not give it a justice, but you can open my game in fullscreen and see what I am referring to.
The buttons have a fixed width and height, which scales if I resize the window. It works well if I scale down - the footer maintains its 20% width, the buttons remain themselves in the center of it and scale down. However, if I go fullscreen on PC, the same process goes the other way around - the footer remains its 20%, the buttons keep their relative positions, but scale up, way over initial size.
In most of my views I keep the relative position of the elements as anchors or sort of guide where the element should position itself. However, when the scale factor goes over 1.0, the elements can easily go out of screen:
In GLSL language there is a clamp function, which clamps the value between further values, e.g. between 0 and 1. Is there a way to achieve a similar functionality with the elements? For example, to clamp its scale between 0.5 and 1.0. In other words, âHey, element, I donât mind you to scale, but do not scale over 1.0 if you are growingâ.
I also tried to experiment with keeping positions relative to the center/top/bottom of the screen, but it didnât go well. In the last screen, for example, the Back button is relative the bottom of the screen and not part of the leaderboard, but it overlaps it, because leaderboard element is relative to the center of the screen.
How do you handle scaling?