This?
var distance = this.entity.getPosition().distance(player.getPosition());
if (distance > 1 && distance < 10) {
// look at the player (probably your model need to be rotated 180 degrees)
var player=this.app.root.findByName(name of the player entity);
this.entity.lookAt(player.getPosition());
// move forward (if the rigidbody is kinematic)
this.entity.translateLocal(0,0,-0.1);
it says, Getposition is undefined
That is because you use the variable player already in your first line, so you have to define the variable before the first line, like how I did it below. In addition, you have to change the name of the player enitity in the findByName
function as you have named it in your scene.
var player = this.app.root.findByName('name of the player entity');
var distance = this.entity.getPosition().distance(player.getPosition());
if (distance > 1 && distance < 10) {
// look at the player (probably your model need to be rotated 180 degrees)
this.entity.lookAt(player.getPosition());
// move forward (if the rigidbody is kinematic)
this.entity.translateLocal(0,0,-0.1);
}
1 Like
lol⌠it says it cant read root property
Can you show me your code please? Also where did you add the code? Is it on the correct place inside the update function, or did you add it random in your script again?
i set it as a different script and attached onto the Neighbor an the Eye
i have 2 models
if you want i will get rid of those scripts and add into the update function
If you want a new script then create a new script and donât remove the code that is in the new script. The initialize function and the update function are almost always required. Place your code inside the update function. I advise you to find out what the initialze function and update function are needed for so you can understand why it doesnât work this way. Also you need to copy and past all code. Included the last bracket. Right now its missing in your âscriptâ. Every bracket that is missing will broke your code.
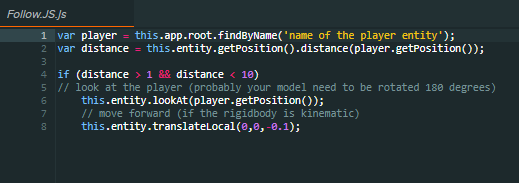
so keep the extra script, but paste it into the Update Function ?
Yes. Better remove this script and make a new one again.
do i attach this script to the Eye and the Neighbor
I donât know what is âthe Eyeâ, but you have to attach the script to only one entity.
i have an eye entity that follows the player, too
You can attach the script to every entity that has to chase the player.
this is from the update function to the end
// HERE START YOUR UPDATE FUNCTION // update code called every frame
Movement.prototype.update = function(dt) {
var player = this.app.root.findByName('name of the player entity');
var distance = this.entity.getPosition().distance(player.getPosition());
if (distance > 1 && distance < 10) {
// look at the player (probably your model need to be rotated 180 degrees)
this.entity.lookAt(player.getPosition());
// move forward (if the rigidbody is kinematic)
** this.entity.translateLocal(0,0,-0.1);**
**}**
// Switch weapon
if (this.app.keyboard.isPressed(pc.KEY_1) || this.app.keyboard.isPressed(pc.KEY_Q)) {
this.flashlight.enabled = true;
this.pistol.enabled = false;
this.menu.enabled = false;
}
if (this.app.keyboard.isPressed(pc.KEY_2) || this.app.keyboard.isPressed(pc.KEY_E)) {
this.flashlight.enabled = false;
this.pistol.enabled = true;
this.menu.enabled = false;
}
if (this.app.keyboard.isPressed(pc.KEY_M)) {
this.flashlight.enabled = false;
this.pistol.enabled = false;
this.menu.enabled = true;
}
// If a camera isn't assigned from the Editor, create one
if (!this.camera) {
this._createCamera();
}
var force = this.force;
var app = this.app;
// Get camera directions to determine movement directions
var forward = this.camera.forward;
var right = this.camera.right;
// movement
var x = 0;
var z = 0;
// Use W-A-S-D keys to move players
// Check for key presses
if (app.keyboard.isPressed(pc.KEY_A) || app.keyboard.isPressed(pc.KEY_LEFT)) {
x -= right.x;
z -= right.z;
}
if (app.keyboard.isPressed(pc.KEY_D) || app.keyboard.isPressed(pc.KEY_RIGHT)) {
x += right.x;
z += right.z;
}
if (app.keyboard.isPressed(pc.KEY_W) || app.keyboard.isPressed(pc.KEY_UP)) {
x += forward.x;
z += forward.z;
}
if (app.keyboard.isPressed(pc.KEY_S) || app.keyboard.isPressed(pc.KEY_DOWN)) {
x -= forward.x;
z -= forward.z;
}
// use direction from keypresses to apply a force to the character
if (x !== 0 && z !== 0) {
force.set(x, 0, z).normalize().scale(this.power);
this.entity.rigidbody.applyForce(force);
}
// update camera angle from mouse events
this.camera.setLocalEulerAngles(this.eulers.y, this.eulers.x, 0);
setTimeout(function() {
// Reload
if (app.keyboard.isPressed(pc.KEY_R)) {
this.rounds = 6;
}
}.bind(this), 3000);
// reload gun magazine
};
Movement.prototype._onMouseMove = function (e) {
// If pointer is disabled
// If the left mouse button is down update the camera from mouse movement
if (pc.Mouse.isPointerLocked() || e.buttons[0]) {
this.eulers.x -= this.lookSpeed * e.dx;
this.eulers.y -= this.lookSpeed * e.dy;
}
};
Movement.prototype._createCamera = function () {
// If user hasn't assigned a camera, create a new one
this.camera = new pc.Entity();
this.camera.setName("First Person Camera");
this.camera.addComponent("camera");
this.entity.addChild(this.camera);
this.camera.translateLocal(0, 0.5, 0);
};
// Function start (recognizable by the script name)
Movement.prototype.onMouseDown = function(event) {
if (this.app.root.findByName('Pistol').enabled === true) {
// shooting code
if (event.button === pc.MOUSEBUTTON_LEFT) {
// shoot
if (this.rounds !== 0) {
var bullet = this.app.root.findByName('Bullet').clone();
this.app.root.addChild(bullet);
bullet.reparent(this.app.root);
bullet.setPosition(this.app.root.findByName('Bullet').getPosition());
bullet.setRotation(this.app.root.findByName('Bullet').getRotation());
bullet.enabled = true;
console.log(bullet);
// update magazine
this.rounds = this.rounds - 1;
}
}
}
// Function end
};
If you add this to your âplayerâ script instead of a new script that you attach to your âenemyâ, this will not work.
Also, you still need to change this line with the exact same name of the player entity in your scene.
so i attached it to the player. and i have fixed that, now what.
now do i attach a new script?
Where do you read that you have to add it to your player script? If you are creating an enemy that is following your player you only have to add it to a script that is attached to your enemy.